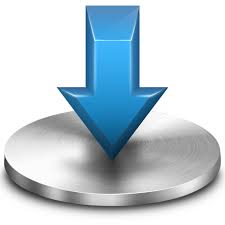
# fails test if `farkle.score(got) != want` Self.assertEqual(farkle.score(got), want) In your case doctest may work better (since you've written examples already that only have to be tweaked slightly to use doctest), but if you start writing more intense functions, it's important to be able to grow your testing suite appropriately. I'm a big fan of the unittest module for writing tests. # then inside the loop we're looking at here.ĭie_score += single_values.get(die, 0) * countįinally at the end of our loop (just before exiting it), we add the single die_score to the roll-wide score.
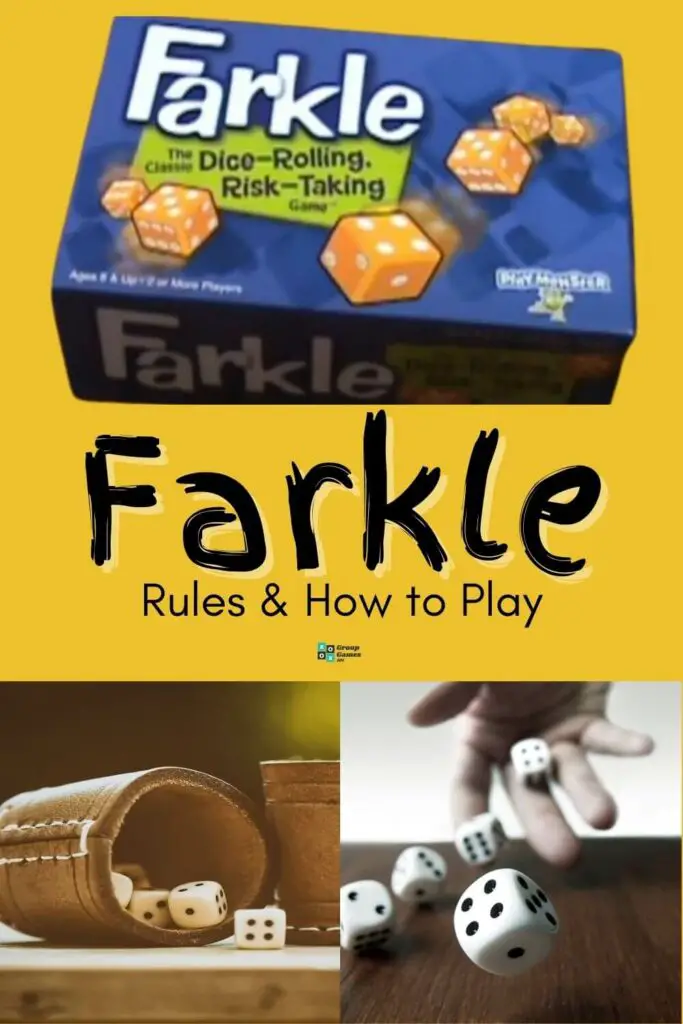
In fact we can factor some of that out to drop the if block. This should handle triplets and quadruplets of any value, and removes from count the number of dice consumed by the grouping. Let's use that! for die, count in ems():ĭie_score += die * 2000 if die = 1 else die * 200ĭie_score += die * 1000 if die = 1 else die * 100 We already know that the triple value for each number is 100 * number, except for 1 which is 100 * 10 * number. If (die = 5 and count != 3): score += (count * 50)įirst of all, avoid these repeated if statements. This should be: for die, count in enumerate(dice_array, start=1):īut since I switched to using a collections.Counter, we can just do: for die, count in ems(): We'll stop here for just long enough to mention that enumerate takes a keyword argument start that lets you tell it where to start counting from. Let's look: for i, count in enumerate(dice_array): Your counting loop isn't a whole lot better.
Rules for farkle dice game code#
counts will throw a KeyError while counts.get(non_rolled_die, 0) will act like your current code does.

It builds a dict-like object, a collections.Counter that has the counts for any dice present in the roll. That might be fast, but it's certainly ugly. This is wonky because it buids a fixed-size list, then plays with values-as-list-indexes to get a count. # as an aside, this isn't an array, it's a list. Let's take a look at a couple examples of low-level work in your code: dice_array = * sides Print(str(score(roll)) + " : " + str(roll))Ĭode is "pythonic" when it expresses its intention clearly, is easy to read or even looks like pseudo-code, and uses as little low-level garbage as possible. Roll = input("Enter 6 values (1-6) separated by a space: ") If (dice = 5 and count != 3): score += (count * 50) Player would choose which die to 'bank' and which to re-rollĭice = i + 1 #this makes it easier to keep track of the die we're on #add up the number appearances of each die roll and store it in the tableīased on the above scoring, determine the MAXIMUM score in actual Farkle the

#create a table to hold the count of each die roll All scoring dice must be rolled in a single turn (i.e. Doubles score nothing (unless a 1 or a 5) as above

Quadruple of any non-1 number: Double the triple score Triple of any non-1 number: 100 x number showing Any and all tips are most welcome! """Ĭalculate a Farkle score using the traditional scoring method, which is: I'd like to hear from the community on how I could make it better and more Pythonic. While the results are correct I don't feel it's a very Pythonic implementation. I've written the following function on Python (3.3) to take a list of 6 integers and return the maximum score for a game of Farkle.
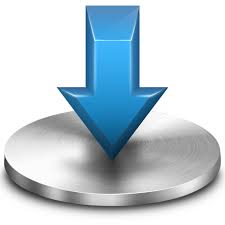